Transposition Systems.
Aim
This page should enable you to develop a tool for cracking any kind of code which works by breaking the plaintext up into blocks and then shuffling letters within those blocks.
Use this tool on ciphers which have the same letter frequency distribution as standard English but appear to be a jumbled mess. If you have a crib (word or two that you are expecting to be in the message) then this could be vital for finding the "anagram" used on the letter block.
It is common practice to pad the message with a few extra letters so that the plaintext can be split exactly into blocks that are all the same length. Thus you need to know how long the message is and what the prime factors of its length are.
The same program can be used to encipher or decipher messages.
Adding text boxes.
Get the worksheet into Design Mode by clicking on the Design Mode toggle.
Now click on the Text Box object to select it.
|
Drag and drop two text boxes onto your work book. These need to be pretty big to make life easier later on.
Your workbook should look like the image below.
Make sure that you save your workbook after every change; especially if the change works.
|
|
Now select a command button from the object menu. Three of these are needed to do various jobs.
Right click on each button to change its caption.
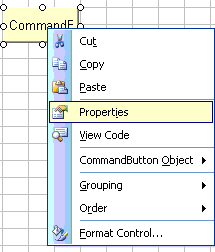
|
The buttons need to be called:
Clean n Case
Length etc.
Shuffle
|
The VBA code now needs to be entered for each command button. To do this you use the VBA editor. The easiest way to summon this is to double left click on an object whilst in design mode.
The cursor is automatically positioned in the correct place for adding VBA code to a control.
Copy and paste the code below into the editor.
Make sure that you don't duplicate the top and bottom lines as they are provided automatically by the editor.
Once you have entered the code you must save the changes you have made. Then reurn to your workbook and exit from design mode. Design mode is entered or left by left clicking its toggle switch.
|
|
|
|
|
|
CLEAN n CASE Button
This button takes a message from the top text box, strips out the punctuation and converts it to uppercase. The result is returned to the upper textbox.
|
Private Sub CommandButton1_Click()
newStr = ""
newStr1 = ""
sourceStr = TextBox1.Value
newStr = Format(sourceStr, ">")
For i = 1 To Len(sourceStr)
If (Asc(Mid(newStr, i, 1)) >= 65) Then
If (Asc(Mid(newStr, i, 1)) <= 90) Then
newStr1 = newStr1 + Mid(newStr, i, 1)
End If
End If
Next i
TextBox1.Value = newStr1
End Sub
|
Format(sourceStr,">") uses an inbuilt VBA function to shift the message to uppercase.
The for loop then filters out anything with an ASCII code less than 65 or greater than 91. The ASCII codes of the uppercase alphabet run from 65 to 90 inclusive.
The cleaned up message is then sent back to the top textbox.
|
Length etc. Button
This button writes the length of the cleaned up plaintext string into cell r18.
It works out the prime factors of this value and writes them to cells s18-s30 or so on.
|
Private Sub CommandButton2_Click()
Dim number1 As Long
Dim trial As Long
Dim rowcounter As Integer
sourceStr = TextBox1.Value
Worksheets("Sheet1").Cells(7, 18).Value = Len(sourceStr)
For n = 0 To 20
Worksheets("Sheet1").Cells(7 + n, 19).Value = ""
Next n
trial = 2
rowcounter = 0
number1 = Worksheets("Sheet1").Cells(7, 18).Value
If (number1 > 1) Then
Worksheets("Sheet1").Cells(7 + rowcounter, 19).Value = number1
Do
If (number1 Mod trial = 0) Then
Worksheets("Sheet1").Cells(7 + rowcounter, 19).Value = trial
number1 = number1 / trial
rowcounter = rowcounter + 1
Worksheets("Sheet1").Cells(7 + rowcounter, 19).Value = number1
Else
trial = trial + 1
End If
Loop Until trial = number1
End If
End Sub
|
The Dim statements are used to set up variables for the factorisation process.
The first for loop clears any debris from previous factorisations.
The do loop then tries trial divisors into the length value. If one is found then it is printed into the correct cell and the process continues.
The loop is slightly inefficient as it could stop at a lower value.
|
Shuffle Button.
|
Private Sub CommandButton3_Click()
Dim shuffBlock As Integer
sourceStr = TextBox1.Value
newStr = ""
shuffBlock = Worksheets("Sheet1").Cells(11, 6).Value
If (Len(sourceStr) Mod shuffBlock = 0) Then
For n = 0 To (Len(sourceStr) / shuffBlock) - 1
shuffStr = Mid(sourceStr, 1 + n * shuffBlock, shuffBlock)
For m = 1 To shuffBlock
letter = Mid(shuffStr, Worksheets("Sheet1").Cells(13, 1 + m).Value, 1)
newStr = newStr + letter
Next m
Next n
End If
TextBox2.Value = newStr
End Sub
|
The length of the block to shuffle is read in. This must be a divisor of the length of the plaintext.
The plaintext is then broken up into blocks of this length.
Each block is then shuffled according to the rule in the cells of row 13. The number in the cell is the place in the plaintext block that the letter going into that position is drawn from.
The shuffled blocks are then stuck back together and sent to the lower textbox.
Test your system very carefully with simple messages to check that it behaves exactly as it should.
|
|
|
last updated 12th September 2011